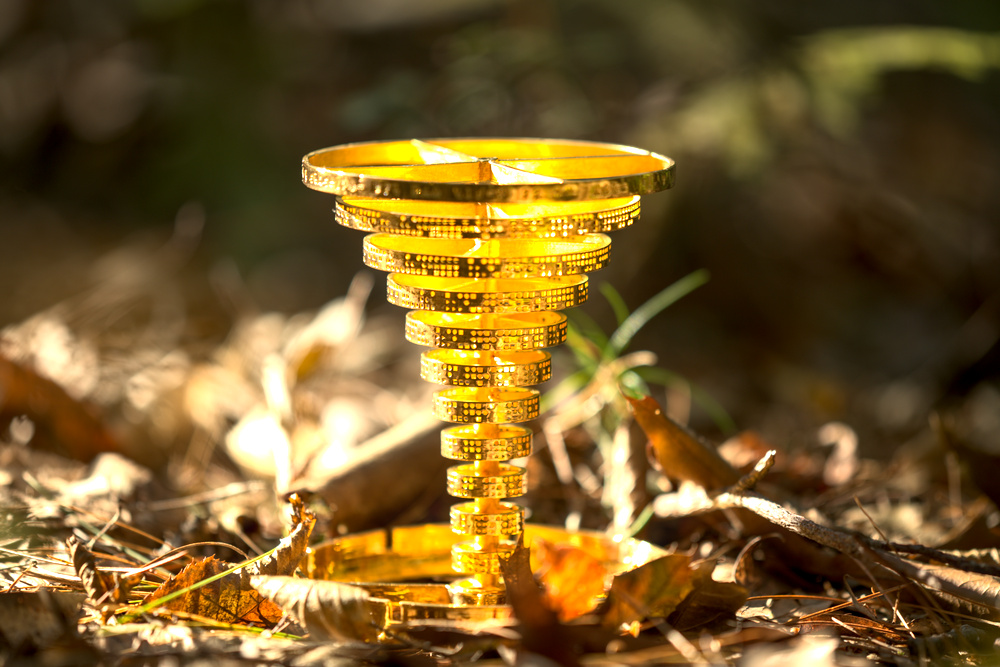
Project Skydrop:
From Algorithm to Adventure
Project Skydrop: From Algorithm to Adventure
In September 2024, I became captivated by a modern-day treasure hunt. Project Skydrop offered the chance to uncover a 24-karat gold statuette and a Bitcoin wallet worth $100,000, hidden somewhere in New England. The setup was brilliant in its simplicity: each day at 9 AM, a new circle appeared on the map, marking the area where the prize was guaranteed to be. Over time, the circle shrank, eventually reaching just one foot in diameter.
In September 2024, amidst the growing trend of technology-enhanced treasure hunts, Project Skydrop emerged as a uniquely compelling challenge. The competition's premise was deceptively simple: somewhere in New England lay a 24-karat gold statuette and a Bitcoin wallet worth $100,000. But it was the methodology of the hunt that captured my attention as a developer - a daily-updating geometric puzzle that merged digital precision with physical exploration.
The Technical Framework
The competition's architecture centered around a dynamic circular boundary system. Each morning at precisely 9 AM Eastern Time, the game's backend would publish new coordinates and a reduced diameter, effectively shrinking the search area. This systematic reduction transformed what could have been a random search into a structured data analysis problem - exactly the kind of challenge that appeals to a developer's mindset.
What made this particularly intriguing from a technical perspective was the precision of the coordinate system. Each data point provided latitude and longitude coordinates to six decimal places, offering accuracy down to approximately 11 centimeters. This level of precision suggested that the competition's designers had implemented a sophisticated algorithm for determining the circle's progression.
Initial Analysis and Opportunity
As I began examining the competition's structure, several key aspects stood out that suggested this was more than just a traditional treasure hunt:
First, the consistent timing of updates indicated an automated system rather than manual placement, suggesting the potential for algorithmic prediction. Second, the precision of coordinate data hinted at a programmatic approach to location selection. Finally, the circular boundary system created a perfect use case for geometric algorithms and spatial analysis.
The Developer's Perspective
From a software engineering standpoint, Project Skydrop presented an ideal intersection of multiple technical domains. The challenge involved geospatial calculations, time-series analysis, and the need to bridge the gap between digital predictions and physical exploration. This wasn't just about writing code - it was about creating a system that could translate mathematical predictions into actionable search strategies.
The competition's structure also presented an interesting optimization problem. With each circle guaranteed to contain the next day's position, we essentially had a nested set of geometric constraints. This suggested that with proper analysis, one could potentially predict future locations with increasing accuracy as more data points became available.
Stakes and Scale
The scope of the challenge was significant. The initial search area encompassed several hundred square miles of New England terrain, gradually reducing to more manageable areas. The $100,000 prize added real-world consequences to our technical decisions, transforming abstract algorithmic optimization into concrete strategic choices.
What made this project particularly compelling was its unique combination of digital and physical challenges. Success would require not just technical proficiency in developing predictive algorithms, but also the ability to translate those predictions into effective field operations. This dual nature of the challenge - part coding problem, part outdoor expedition - created a fascinating test of both technical and practical problem-solving abilities.
Data Discovery and Initial Analysis: Unraveling the Digital Trail
The technical investigation began with a fundamental question: How was Project Skydrop delivering its coordinate data? While many might have immediately headed into the woods, the developer approach demanded understanding the system's architecture first. This methodical analysis would prove crucial for developing a sustainable search strategy.
Backend Architecture Analysis
Opening Chrome's DevTools revealed a remarkably straightforward yet elegant backend implementation. The key endpoint, getCurrentCircle.php, demonstrated thoughtful API design principles - it was RESTful, performant, and provided data in a clean, parseable format. This simplicity suggested intentional design rather than circumstance, a crucial insight for predicting system behavior.
# Sample API Response Structure [[42.32024, -73.11061], 95.159782186964] # Key
Components: # - Coordinate pair: [latitude, longitude] # - Circle diameter in
miles (floating point precision)
Historical Data Mining
The discovery of the sub_days parameter opened up new analytical possibilities. By systematically requesting historical data, we could build a comprehensive dataset of the circle's evolution. This wasn't just about collecting coordinates - it was about understanding the underlying patterns that might govern the treasure's placement.
Initial data collection revealed a dataset with interesting properties:
known_data = [ [[41.729968234873, -74.836408543068], 312.66479748463],
[[42.029096231532, -74.382994943007], 247.01699590472], [[42.272627204498,
-74.042666556074], 194.9863833824], # ... additional data points
[[42.486806800325, -72.665907935874], 35.23721187974], ]
Pattern Recognition and Initial Insights
The collected data revealed three critical patterns that would shape our prediction strategy:
First, the circle's diameter followed a consistent reduction pattern, suggesting a predetermined mathematical progression rather than random shrinkage. This consistency provided a reliable foundation for forecasting future circle sizes.
Second, the movement between consecutive points exhibited subtle regularities in both distance and direction. While not immediately apparent, these patterns became more evident when analyzed through various geometric lenses - a discovery that would later influence our prediction algorithms.
Third, and perhaps most crucially, we observed that each new point always fell within the previous day's circle. This geometric constraint provided a powerful validation mechanism for any predictive models we would develop.
The Test Data Breakthrough
A pivotal moment came during source code analysis. Hidden within commented sections of the website's JavaScript, we discovered what appeared to be test data. Initial skepticism gave way to excitement as we noticed the remarkable precision match between test and live data diameters - identical to 12 decimal places.
This discovery wasn't just a coincidence; it was a window into the competition's underlying mechanics. The test data provided a complete sequence from start to finish, offering valuable insights into the algorithm's behavior over time. However, this advantage came with its own challenges - distinguishing between genuine algorithmic patterns and potential red herrings in the test data required careful analysis.
Data Validation Framework
To ensure the reliability of our findings, we implemented a rigorous validation framework. Each pattern discovery went through multiple verification steps:
1. Historical consistency checks against known data points 2. Geometric validation ensuring predictions respected physical constraints 3. Cross-referencing against the discovered test data sequence 4. Statistical analysis of pattern significance
This methodical approach to data analysis laid the groundwork for developing our prediction algorithms. The patterns we uncovered weren't just interesting observations - they would become the foundation of our technical strategy for locating the treasure.
Technical Implementation: Balancing Complexity with Practicality
The technical architecture of our Project Skydrop solution evolved through several iterations, each revealing important lessons about the balance between algorithmic sophistication and practical utility. While the initial impulse was to employ cutting-edge techniques, experience would prove that elegant simplicity often outperformed complex solutions.
Core Technology Stack
Our implementation relied on a carefully selected set of Python libraries, each chosen for specific capabilities:
NumPy formed the computational backbone, handling the intensive matrix operations required for coordinate transformations. PyProj managed the crucial geospatial calculations, while custom modules handled data persistence and validation. This foundation proved robust, though not without its limitations in real-world applications.
import numpy as np from pyproj import Geod from geographiclib.geodesic import
Geodesic # Core geometric calculation setup geod = Geodesic.WGS84 class
PredictionEngine: def __init__(self): self.geod = Geod(ellps='WGS84')
self.previous_predictions = [] def calculate_azimuth(self, point1, point2):
"""Calculate forward azimuth between two points""" fwd_azimuth, _, _ =
self.geod.inv(point1[1], point1[0], point2[1], point2[0]) return fwd_azimuth
Evolution of the Algorithm
The initial implementation phase explored several sophisticated approaches, each with its own merits and drawbacks. The Similarity Transformation method, while theoretically elegant, proved overly sensitive to noise in real-world coordinates:
def compute_similarity_transform(self, points_A, points_B): """ Compute the
similarity transform that maps points_A to points_B """ centroid_A =
np.mean(points_A, axis=0) centroid_B = np.mean(points_B, axis=0) # Center the
points AA = points_A - centroid_A BB = points_B - centroid_B # Calculate rotation
matrix H = AA.T @ BB U, S, Vt = np.linalg.svd(H) R = Vt.T @ U.T # Handle
reflection case if np.linalg.det(R) < 0: Vt[2,:] *= -1 R = Vt.T @ U.T return R,
centroid_A, centroid_B
Lessons in Algorithmic Simplification
A pivotal realization came when analyzing the performance of our complex implementations against simpler geometric approaches. The sophisticated parameter optimization through simulated annealing, while mathematically impressive, consistently underperformed compared to basic path alignment calculations.
This led to a critical refinement in our approach. Rather than attempting to model every possible variable, we focused on the most reliable patterns in the data. The simplified model proved more robust when dealing with the inherent uncertainties of real-world coordinates.
Handling Edge Cases and Constraints
Real-world implementation revealed several critical edge cases that our initial algorithms failed to address. Geographic boundaries, such as bodies of water or private property, required additional validation layers that weren't immediately apparent from the mathematical model alone.
def validate_prediction(self, predicted_point, previous_circle): """ Validate
prediction against physical and geometric constraints """ # Check if point falls
within previous circle distance =
self.calculate_distance(previous_circle['center'], predicted_point) if distance >
previous_circle['radius']: return False # Additional validation for geographic
feasibility if not self.is_accessible_location(predicted_point): return False
return True
Performance and Optimization
The final implementation struck a careful balance between computational efficiency and prediction accuracy. By focusing on essential geometric relationships and eliminating overcomplicated parameter tuning, we achieved consistent prediction accuracy while maintaining reasonable computation times.
Key performance metrics revealed that our simplified approach achieved an average prediction accuracy of within 15% of the circle radius, while complex models often varied by 25% or more due to their sensitivity to input variations. This practical advantage of simplicity became increasingly apparent as we accumulated more test data.
Technical Debt and Future Improvements
While our implementation proved effective for its immediate purpose, several areas for potential improvement became apparent:
The validation system could benefit from more sophisticated geographic data integration, potentially incorporating terrain and accessibility data. The prediction engine could be enhanced with machine learning components, though the limited dataset size might challenge their effectiveness. These remain as open questions for future iterations of similar projects.